[LC] 2. Add Two Numbers
TechYou are given two non-empty linked lists representing two non-negative integers. The digits are stored in reverse order, and each of their nodes contains a single digit. Add the two numbers and return the sum as a linked list.
You may assume the two numbers do not contain any leading zero, except the number 0 itself.
Example 1:
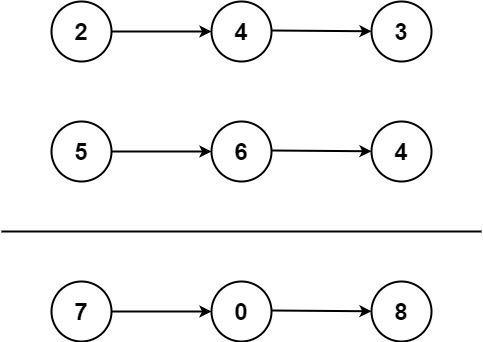
Input: l1 = [2,4,3], l2 = [5,6,4] Output: [7,0,8] Explanation: 342 + 465 = 807.
Example 2:
Input: l1 = [0], l2 = [0] Output: [0]
Example 3:
Input: l1 = [9,9,9,9,9,9,9], l2 = [9,9,9,9] Output: [8,9,9,9,0,0,0,1]
Constraints:
- The number of nodes in each linked list is in the range
[1, 100]
. 0 <= Node.val <= 9
- It is guaranteed that the list represents a number that does not have leading zeros.
Approach
Approach is simple. Just looking all of the list pair of list1 and list2, and calculate sum = (list1.val + list2.val + prev_carry)%10 and carry = (list1.val + list2.val + prev_carry) // 10. Loop both list first, and list1, list2 consequentially. If carry is 1 at the last, we should add it to the last element.
# Definition for singly-linked list.
# class ListNode:
# def __init__(self, val=0, next=None):
# self.val = val
# self.next = next
class Solution:
def addTwoNumbers(self, l1: ListNode, l2: ListNode) -> ListNode:
root = ListNode(0)
head = root
s,c = 0,0
while l1 and l2:
s,c = (l1.val+l2.val+c)%10, (l1.val+l2.val+c)//10
root.next = ListNode(s)
l1,l2,root = l1.next, l2.next, root.next
while l1:
s,c = (l1.val+c)%10, (l1.val+c)//10
root.next = ListNode(s)
l1, root = l1.next, root.next
while l2:
s,c = (l2.val+c)%10, (l2.val+c)//10
root.next = ListNode(s)
l2, root = l2.next, root.next
if c != 0:
root.next = ListNode(c)
return head.next
Time/Space Complexity: O(N)
Submission
Runtime: 88 ms, faster than 10.65% of Python3 online submissions for Add Two Numbers.
Memory Usage: 14.4 MB, less than 12.54% of Python3 online submissions for Add Two Numbers.